Radio buttons allow the user to select one option from a set. You should use radio buttons for optional sets that are mutually exclusive if you think that the user needs to see all available options side-by-side. If it's not necessary to show all options side-by-side, use a spinner instead.
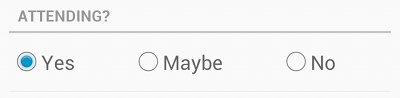
To create each radio button option, create a RadioButton
in your layout.
However, because radio buttons are mutually exclusive, you must group them together inside a
RadioGroup
. By grouping them together, the system ensures that only one
radio button can be selected at a time.
Responding to Click Events
When the user selects one of the radio buttons, the corresponding RadioButton
object receives an on-click event.
To define the click event handler for a button, add the android:onClick
attribute to the
<RadioButton>
element in your XML
layout. The value for this attribute must be the name of the method you want to call in response
to a click event. The Activity
hosting the layout must then implement the
corresponding method.
For example, here are a couple RadioButton
objects:
<?xml version="1.0" encoding="utf-8"?> <RadioGroup xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="wrap_content" android:orientation="vertical"> <RadioButton android:id="@+id/radio_pirates" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/pirates" android:onClick="onRadioButtonClicked"/> <RadioButton android:id="@+id/radio_ninjas" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/ninjas" android:onClick="onRadioButtonClicked"/> </RadioGroup>
Note: The RadioGroup
is a subclass of
LinearLayout
that has a vertical orientation by default.
Within the Activity
that hosts this layout, the following method handles the
click event for both radio buttons:
public void onRadioButtonClicked(View view) { // Is the button now checked? boolean checked = ((RadioButton) view).isChecked(); // Check which radio button was clicked switch(view.getId()) { case R.id.radio_pirates: if (checked) // Pirates are the best break; case R.id.radio_ninjas: if (checked) // Ninjas rule break; } }
The method you declare in the android:onClick
attribute
must have a signature exactly as shown above. Specifically, the method must:
Tip: If you need to change the radio button state
yourself (such as when loading a saved CheckBoxPreference
),
use the setChecked(boolean)
or toggle()
method.